Use MaximoPlus to add search forms and utilize Maximo domains through a list of values.
This is a part two of series, please read first the https://maximoplus.com/blog/getting-started-with-maximoplus/.
Search is one of the basic functionalities in Maximo. In MaximoPlus, we use QBE Sections for that. They are entirely equivalent to the Advanced Search Forms in Maximo itself and use the same Maximo QBE (Query by Example).
Create the search screen in screens/POQbeSection.js file, and paste the following:
import React, { Component } from "react";
import { QbeSection } from "../components/Section";
import HeaderActionButtons from "../components/HeaderActionButtons";
export default class extends Component {
static navigationOptions = ({ navigation }) => {
const buttons = navigation.getParam("qbeButtons");
const iconMap = { clear: "md-close", search: "md-checkmark" };
return {
headerTitle: "Search POs",
headerRight: <HeaderActionButtons buttons={buttons} icons={iconMap} />
};
};
render() {
return (
<QbeSection
container="pocont"
columns={["ponum", "description", "status", "shipvia"]}
navigateTo="List"
/>
);
}
}
Once you finished editing the new file, don't forget to change the MainTabNavigation.js to include it:
const QBESectionStack = createStackNavigator(
{ QBESection: POQbeSectionScreen },
config
);
As you can see from above, the QbeSection component looks almost the same as the Section we introduced before. It has one new attribute, navigateTo where you specify which page should be open after the search finishes.
You can spot one more difference in the navigation options. QBE section renders two buttons in the navigation header: one for performing the search, and another for clearing the QBE. You can read more about navigation header buttons on https://reactnavigation.org/docs/en/header-buttons.html.
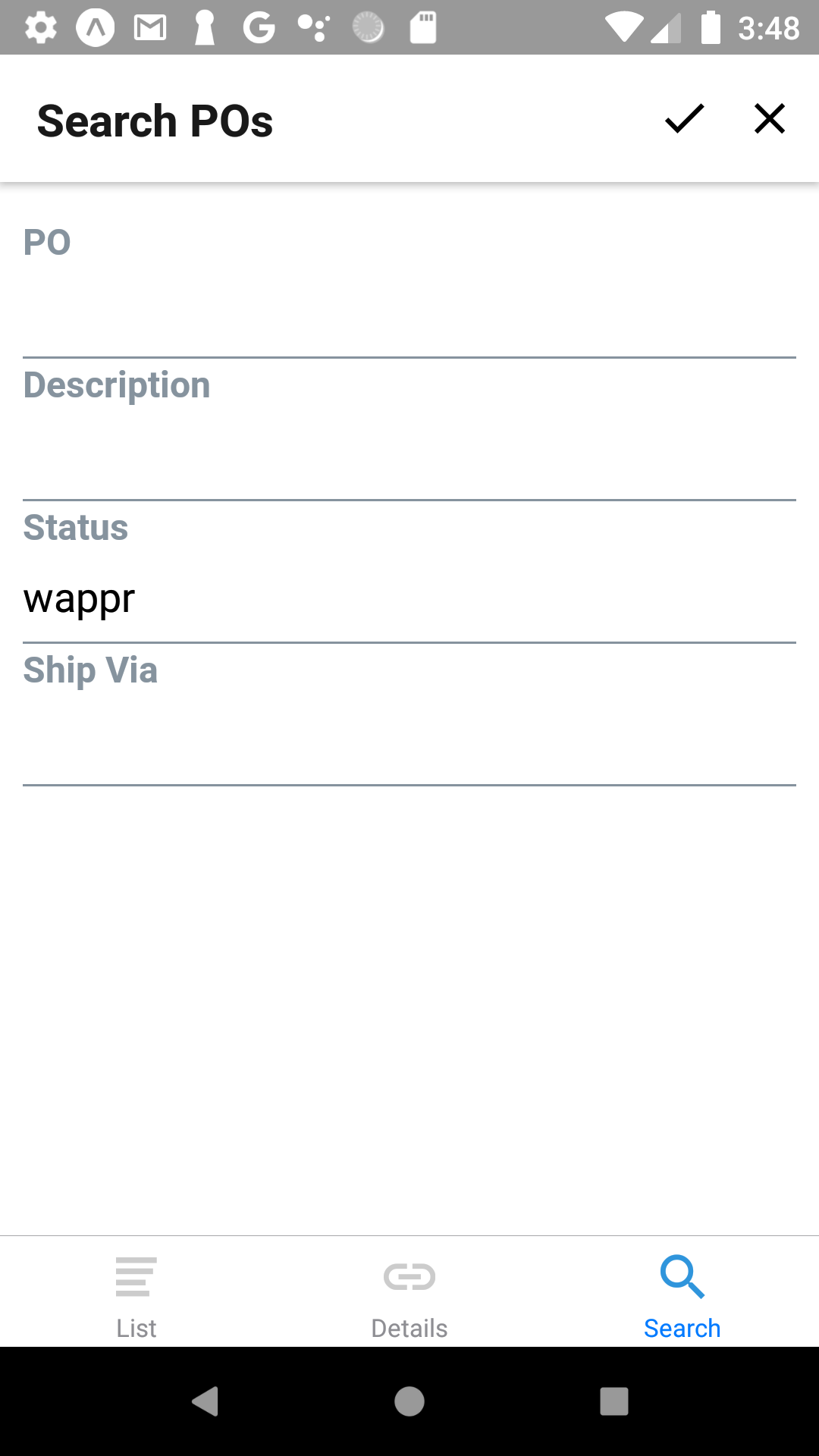
You can try to enter the search criteria as above and perform the search by clicking on the check icon.
Metadata
We need briefly to talk about metadata before we introduce the value lists. In MaximoPlus, metadata is information about the attributes of the Mbo: its label, data type, description, domain id. Most of the time, the metadata comes automatically from Maximo, and this is how the captions were displayed in the Qbe Section above, even though we didn't specify them.
In addition to the automatic metadata, we can add the metadata in the definition of the component. For example:
render() {
return (
<QbeSection
container="pocont"
columns={["ponum", "description", "status", "shipvia"]}
navigateTo="POList"
metadata={{
SHIPVIA: {
hasLookup: true,
listTemplate: "qbevaluelist"
},
STATUS: {
hasLookup: true,
listTemplate: "qbevaluelist"
}
}}
/>
);
For this QBE Section, we appended the metadata attributes hasLookup and listTemplate to the attributes SHIPVIA and STATUS.
MaximoPlus template uses the metadata to customize any field behavior. The most important ones are the value lists.
Value Lists
The above example specifies the lookups on the SHIPVIA and STATUS fields of the QBE Section. If we define the hasLookup attribute, the Section adds the search to the text field. Since the value lists will show as lists in a separate screen, we need to define the listTemplate property as well. The valuelist and qbevaluelist are the built-in templates; you don't need to change the listTemplates.js file; this is for the reference only:
valuelist: ({ VALUE, DESCRIPTION }) => (
<ListItem title={VALUE} subtitle={DESCRIPTION} />
),
qbevaluelist: ({ VALUE, DESCRIPTION, _SELECTED }) => (
<ListItem
title={VALUE}
subtitle={DESCRIPTION}
leftIcon={
_SELECTED === "Y" ? <Icon name="check" type="font-awesome" /> : null
}
/>
),
You can place the list of values on both the Section and the QbeSection. There is the difference in behavior, same as in Maximo: you can pick only one value for the Section, but you can choose multiple lines in Qbe Section for more complicated searches. That is the reason why we utilize the virtual field _SELECTED, it indicates when the user selects a row.
Change your POQbeSection.js file, as per the example in the metadata section, and try to search for multiple values:
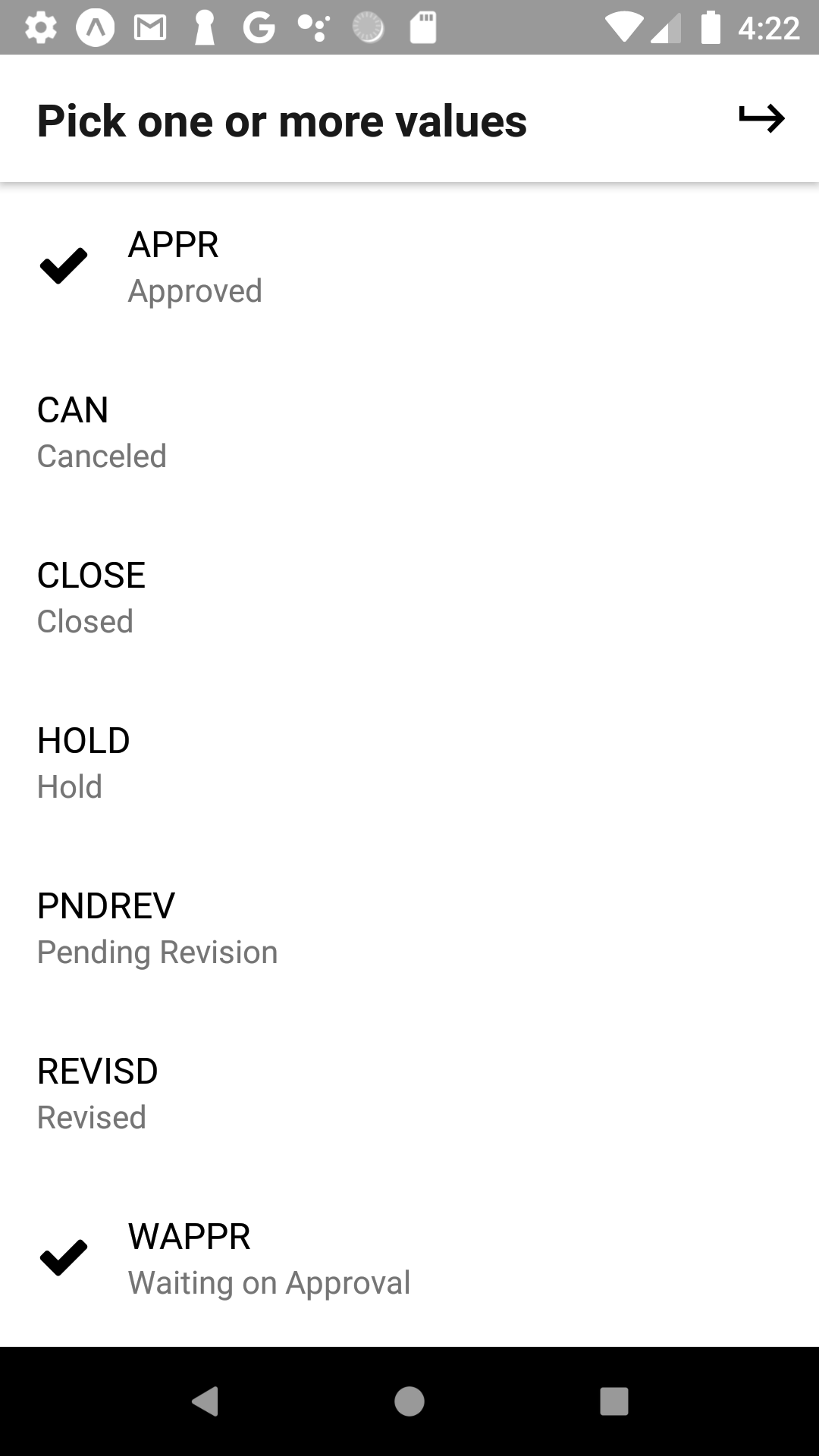
Read the next post in the series to learn more Container concepts, and how to save the MaximoPlus data changes to Maximo.